Using Azure Automation for Event Notifications
Event Notifications are a feature that is a bit underrated in the UEM world. With Event Notifications, you can send details via PUSH to a web server. You can use this information to automate things inside or outside of Workspace ONE UEM.
In this example, we are showing how to use this feature to automate the tagging of devices. Tagging is often used for smart group assignments and normally done via Intelligence. In specific use cases, like Windows Autopilot Hybrid-Join, the Intelligence tagging is too slow and causes in enrollment issues.
For this, we can use an external automation provider that supports webhooks. We are showing this with Azure Automation, but this is just an example of what you can achieve with automations outside of Workspace ONE UEM and Intelligence.
Creating the Azure Automation
First, we start with creating the Azure Automation. For this, please log in to your Azure portal. Open/search for “Automation Accounts”. If you don’t have an Automation Account, please create one. Otherwise, open the existing one and create a new Runbook.
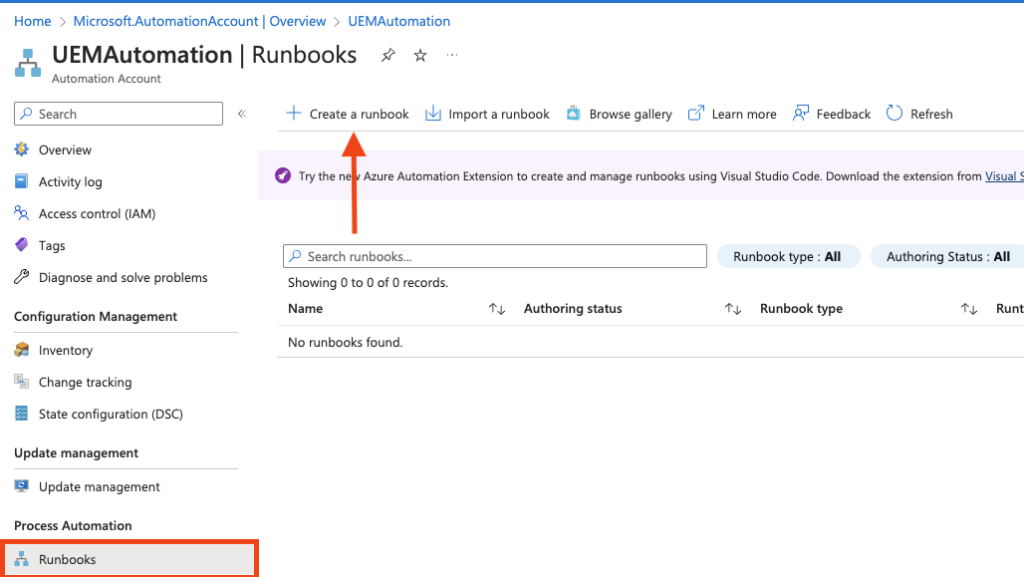
Choose a name and select “PowerShell” as Runbook type and “5.1” as Runtime version.
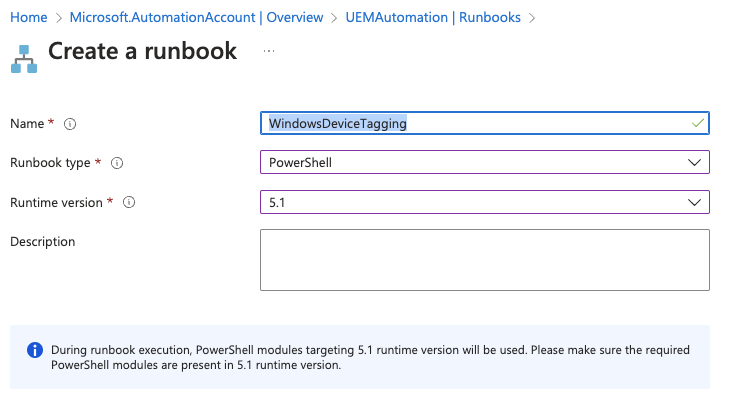
Create the Runbook
The next window that opens is the code editor. Now you can paste the following code:
param
(
[Parameter(Mandatory=$false)]
[object] $WebhookData
)
######################################
# Define UEM Variables
$TagID = "293070" #Select the Tag ID you want to add to the device
$OGName = "ModernManagement" #Select OG name that you want to check for enrollments
######################################
# Get Automation Variables
$APIserver = Get-AutomationVariable -Name 'UEMServer'
$APIClientSecret = Get-AutomationVariable -Name 'ClientSecret'
$APIClientID = Get-AutomationVariable -Name 'ClientID'
$APIOAuthTenant = Get-AutomationVariable -Name 'APIOAuthTenant'
$APIKey = Get-AutomationVariable -Name 'UEMAPIKey'
######################################
# Functions
function Get-UEMOauthToken
{
param(
[string]$APIClientID, #UEM client app ID
[string]$APIClientSecret, #UEM client app secret
[string]$Tenant #UEM client authentication URL - see https://docs.vmware.com/en/VMware-Workspace-ONE-UEM/services/UEM_ConsoleBasics/GUID-BF20C949-5065-4DCF-889D-1E0151016B5A.html for the right URL
)
#Create Header for getting BEARER Token
$headers = @{
'Content-Type' = 'application/x-www-form-urlencoded'
}
#Add the API information to the body
$body = @{
'client_id' = $APIClientID
'client_secret' = $APIClientSecret
'grant_type' = "client_credentials"
}
#run the query against the UEM authentication URL
$response = Invoke-RestMethod $Tenant -Method 'POST' -Headers $headers -Body $body
#extract the bearer token
$token = $response.access_token
$authorizationHeader = "Bearer ${token}"
return $authorizationHeader
}
function Create-UEMAPIHeader
{
param(
[string] $authorizationHeader,
[string] $APIKey,
[string] $ContentType = "json",
[string] $Accept = "json",
[int] $APIVersion = 1
)
#generate Header
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("aw-tenant-code", $APIKey)
$headers.Add("Authorization", $authorizationHeader)
$headers.Add("Accept", "application/$($Accept);version=$($APIVersion)")
$headers.Add("Content-Type", "application/$($ContentType)")
return $headers
}
Function New-JSONBody {
Param([Array]$deviceList)
$arrayLength = $deviceList.Count
$counter = 0
$quoteCharacter = [char]34
$addTagJSON = "{ " + $quoteCharacter + "BulkValues" + $quoteCharacter + " : { " + $quoteCharacter + "Value" + $quoteCharacter + " : [ "
foreach ($currentDeviceID in $deviceList) {
$deviceIDString = Out-String -InputObject $currentDeviceID
$deviceIDString = $deviceIDString.Trim()
$counter = $counter + 1
if ($counter -lt $arrayLength) {
$addTagJSON = $addTagJSON + $quoteCharacter + $deviceIDString + $quoteCharacter + ", "
} else {
$addTagJSON = $addTagJSON + $quoteCharacter + $deviceIDString + $quoteCharacter
}
}
$addTagJSON = $addTagJSON + " ] } }"
Return $addTagJSON
}
######################################
# Script Start
# Get the Bearer token for authentication
$authorizationHeader = Get-UEMOauthToken -APIClientID $APIClientID -APIClientSecret $APIClientSecret -Tenant $APIOAuthTenant
# Create the API Header
$headers = Create-UEMAPIHeader -authorizationHeader $authorizationHeader -APIKey $apiKey
# Convert the webhook data from JSON
$Data = $WebhookData.RequestBody | ConvertFrom-Json
# Get device details
$RESTresponse = Invoke-RestMethod "https://$($APIserver)/API/mdm/devices?searchby=DeviceId&id=$($Data.DeviceID)" -Method 'GET' -Headers $headers
$DevicedetailsTemp = $RESTresponse | Convertto-Json
$DeviceDetails = $DevicedetailsTemp | ConvertFrom-Json
# Check if the device is enrolled in the right OG and is a Windows Device
if($DeviceDetails.LocationGroupName -eq $OGName -and $DeviceDetails.Platform -eq "WinRT")
{
#Create the JSON Body for the API command
$json = New-JSONBody -deviceList $Data.DeviceId
#Tag the device
$RESTresponse = Invoke-RestMethod "https://$($APIserver)/API/mdm/tags/$($TagID)/adddevices" -Method 'POST' -Headers $headers -Body $json
$RESTresponse
}
Please change line 10 and 11 to the required settings.
After you changed the lines, please click “Publish”.
Create the Variables
As you can see in lines 17 to 21, I’m using Automation variables to provide the global API settings.
To configure the variables, please navigate back to the Automation Account and select “Variables”
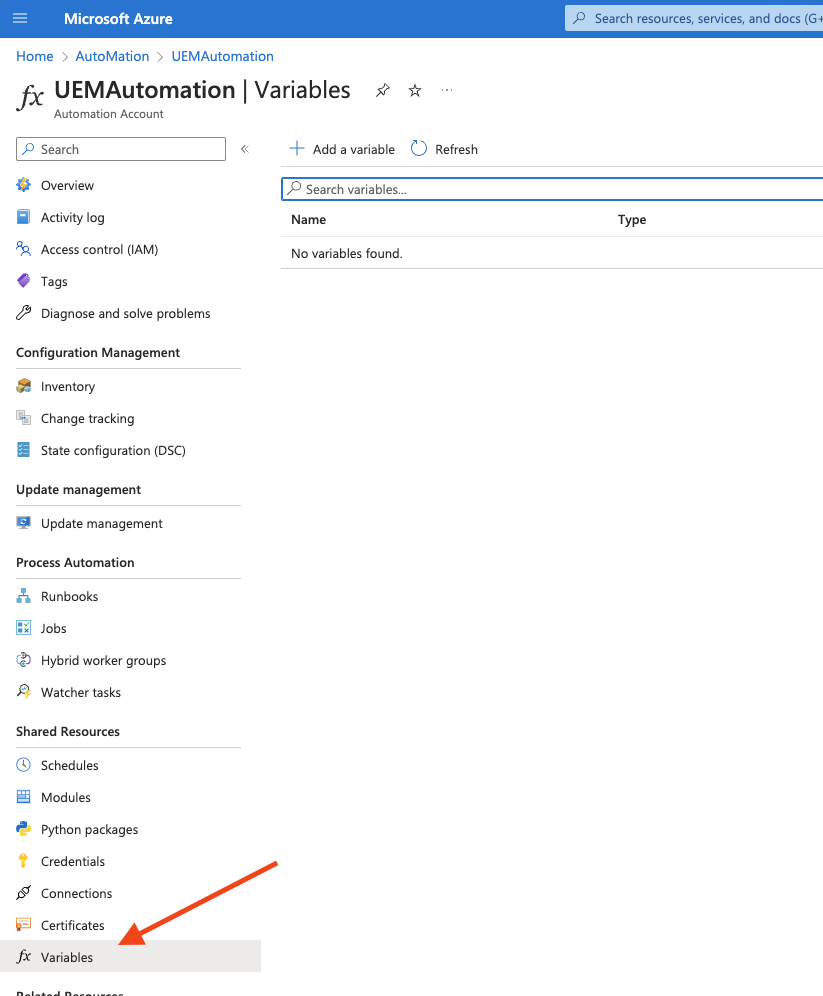
Click “Add a variable” and add all required variables:
- UEMServer
- ClientSecret
- ClientID
- APIOAuthTenant (get the right tenant from here)
- UEMAPIKey
You can also encrypt the values, e.g. for the Client Secret or the UEM API key. This will make sure that no other administrator can view the values.
After you added the variables, it should look like this:

Create the webhook listener
Next, open the runbook again and open “Webhooks” -> click “Add Webhook”.
On the next page, select “create a new webhook” and type in a name.
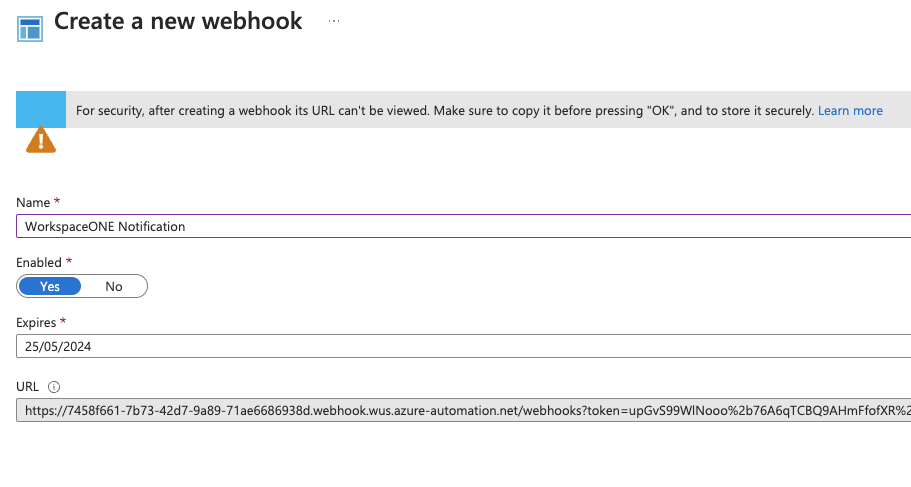
Please copy the listed URL.
Be aware that the URL will be disabled automatically after one year. So, make sure you update the Webhook before the link expires.
We don’t need any additional parameters, so just create the webhook.
Configuration of UEM
After we finished the Azure configuration, we can now create the event notification in Workspace ONE UEM.
Open “All Settings” -> System -> Advanced -> API -> Event Notification
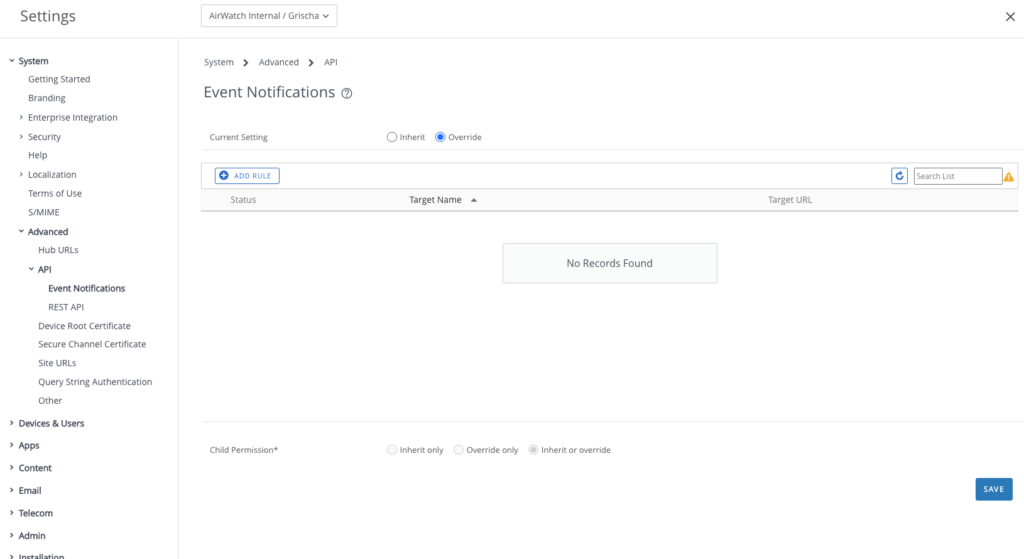
Make sure you have “Override” selected.
Create a new rule and type in a Name – e.g.” Azure Device Tagging” and paste the URL that you copied from the Azure webhook.
We don’t need an authentication, so make sure that the UserName and Password fields are empty. As format, select “JSON” and run a test connection.
If the test was successful, only enable “Device Enrollment”
The next time a device gets enrolled, the UEM Server sends the device information to the Azure Runbook, this Runbook will query the UEM API for the device detailed information. If the device meets all requirements, the script will add a tag to the device.
Empowering customers in client management since 2012.
Empowering customers in modern management since 2018.